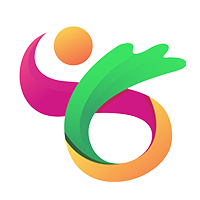
SMTP Mailer API
API SMTP Mailer memungkinkan pengguna untuk mengirim email melalui server SMTP yang dikonfigurasi. Selain itu, Pengguna dapat dengan memudahkan untuk pengiriman email.
Demo: Register-Verification-Email-Demo.zip
Endpoint API (POST)
https://smtp.i-as.dev/api/mailer
Body Header
{
"server": "smtp.example.com",
"port": "465",
"email": "your-email@example.com",
"password": "your-email-password",
"secure": "true",
"to": "recipient@example.com",
"subject": "Test Email",
"text": "This is a test email."
}
CURL
curl -X POST https://smtp.i-as.dev/api/mailer \
-H "Content-Type: application/json" \
-d '{
"server": "smtp.example.com",
"port": "587",
"email": "your-email@example.com",
"password": "your-email-password",
"secure": "false",
"to": "recipient@example.com",
"subject": "Test Email",
"text": "This is a test email."
}'
Response
- Success
Status: 200 OK
Content: Email sending
- Error
Status 400 Bad Request if any field is empty or invalid.
Status 500 Internal Server Error if there is a problem sending email.
Use Javascript
const smtpData = {
server: "smtp.example.com",
port: "587",
email: "your-email@example.com",
password: "your-email-password",
secure: "false",
to: "recipient@example.com",
subject: "Test Email",
text: "This is a test email."
};
async function testSmtpConnection() {
try {
const response = await fetch('https://smtp.i-as.dev/api/mailer', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(smtpData)
});
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
const result = await response.json();
console.log('Response:', result);
} catch (error) {
console.error('Error:', error);
}
}
testSmtpConnection();
Use PHP
<?php
$data = [
'server' => 'smtp.example.com',
'port' => '456',
'email' => 'your-email@example.com',
'password' => 'your-email-password',
'secure' => 'false',
'to' => 'recipient@example.com',
'subject' => 'Test Email',
'text' => 'This is a test email.'
];
$jsonData = json_encode($data);
$ch = curl_init('https://smtp.i-as.dev/api/mailer');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Content-Length: ' . strlen($jsonData)
]);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response: ' . $response;
}
curl_close($ch);
?>